What is a Doughnut Operator?
A doughnut operator, also known as the null coalescing operator, is a binary operator that is used to provide a default value for a nullable variable. It is represented by two question marks (??). The doughnut operator is used to check if the left-hand operand is null. If it is, the right-hand operand is returned. If the left-hand operand is not null, the left-hand operand is returned.
For example, the following code uses the doughnut operator to provide a default value for the name variable:
String name = null;String defaultName ="John Doe";String fullName = name ?? defaultName;
In this example, if the name variable is null, the defaultName variable will be assigned to the fullName variable. If the name variable is not null, the name variable will be assigned to the fullName variable.
The doughnut operator is a useful tool for providing default values for nullable variables. It can help to prevent NullPointerExceptions and make your code more robust.
The doughnut operator was introduced in Java 8. It is supported by all major Java compilers and IDEs.
Doughnut Operator
The doughnut operator, also known as the null coalescing operator, is a binary operator that is used to provide a default value for a nullable variable. It is represented by two question marks (??). The doughnut operator is used to check if the left-hand operand is null. If it is, the right-hand operand is returned. If the left-hand operand is not null, the left-hand operand is returned.
- Null-safety: The doughnut operator helps to prevent NullPointerExceptions by providing a default value for nullable variables.
- Conciseness: The doughnut operator provides a concise way to provide a default value for a nullable variable.
- Readability: The doughnut operator makes code more readable by eliminating the need for lengthy if-else statements to check for null values.
- Versatility: The doughnut operator can be used with any data type, including primitive types, objects, and arrays.
- Extensibility: The doughnut operator can be used to create custom null-safe operators.
- Performance: The doughnut operator is efficient and does not introduce significant overhead.
- Cross-platform: The doughnut operator is supported by all major Java compilers and IDEs.
- Future-proof: The doughnut operator is a modern language feature that is likely to be supported in future versions of Java.
The doughnut operator is a powerful tool that can help to improve the quality of your code. By using the doughnut operator, you can prevent NullPointerExceptions, make your code more concise and readable, and improve its performance.
1. Null-safety
The doughnut operator is a powerful tool for preventing NullPointerExceptions. A NullPointerException is a runtime error that occurs when a program attempts to access a null value. Null values are often used to represent the absence of a value, but they can also be caused by errors in your code. The doughnut operator can help to prevent NullPointerExceptions by providing a default value for nullable variables.
For example, the following code uses the doughnut operator to provide a default value for the name variable:
javaString name = null;String defaultName ="John Doe";String fullName = name ?? defaultName;
In this example, if the name variable is null, the defaultName variable will be assigned to the fullName variable. If the name variable is not null, the name variable will be assigned to the fullName variable.
The doughnut operator is a simple but effective way to prevent NullPointerExceptions. By using the doughnut operator, you can ensure that your code will always have a valid value to work with.
Here are some of the benefits of using the doughnut operator:
- Improved code safety: The doughnut operator can help to prevent NullPointerExceptions, which can lead to program crashes.
- Increased code readability: The doughnut operator can make your code more readable and easier to understand.
- Reduced code complexity: The doughnut operator can help to reduce the complexity of your code by eliminating the need for lengthy if-else statements to check for null values.
The doughnut operator is a valuable tool for any Java developer. By using the doughnut operator, you can improve the safety, readability, and performance of your code.
2. Conciseness
The doughnut operator is a concise way to provide a default value for a nullable variable. This is because it eliminates the need to use an if-else statement to check for null values. For example, the following code uses an if-else statement to check for null values:
javaString name = null;String defaultName ="John Doe";String fullName;if (name == null) { fullName = defaultName;} else { fullName = name;}
The following code uses the doughnut operator to provide a default value for the name variable:
javaString name = null;String defaultName ="John Doe";String fullName = name ?? defaultName;
As you can see, the doughnut operator is a more concise way to provide a default value for a nullable variable. This can make your code more readable and easier to maintain.
The doughnut operator is particularly useful when you are dealing with multiple nullable variables. For example, the following code uses the doughnut operator to provide default values for two nullable variables:
javaString firstName = null;String lastName = null;String fullName = firstName ?? lastName ?? "John Doe";
This code is much more concise than using if-else statements to check for null values.
The doughnut operator is a valuable tool for any Java developer. It can help you to write more concise and readable code. By using the doughnut operator, you can also reduce the number of NullPointerExceptions in your code.
3. Readability
The doughnut operator is a valuable tool for improving the readability of your code. By eliminating the need for lengthy if-else statements to check for null values, the doughnut operator makes your code more concise and easier to understand.
For example, the following code uses an if-else statement to check for null values:
javaString name = null;String defaultName ="John Doe";String fullName;if (name == null) { fullName = defaultName;} else { fullName = name;}
The following code uses the doughnut operator to provide a default value for the name variable:
javaString name = null;String defaultName ="John Doe";String fullName = name ?? defaultName;
As you can see, the doughnut operator is a more concise way to provide a default value for a nullable variable. This can make your code more readable and easier to maintain.
The doughnut operator is particularly useful when you are dealing with multiple nullable variables. For example, the following code uses the doughnut operator to provide default values for two nullable variables:
javaString firstName = null;String lastName = null;String fullName = firstName ?? lastName ?? "John Doe";
This code is much more concise than using if-else statements to check for null values.
The doughnut operator is a valuable tool for any Java developer. It can help you to write more concise and readable code. By using the doughnut operator, you can also reduce the number of NullPointerExceptions in your code.
4. Versatility
The doughnut operator is a versatile operator that can be used with any data type, including primitive types, objects, and arrays. This makes it a powerful tool for handling null values in your code.
- Primitive types
The doughnut operator can be used with primitive types to provide a default value if the variable is null. For example, the following code uses the doughnut operator to provide a default value for the age variable:
java int age = null; int defaultAge = 21; int actualAge = age ?? defaultAge;
In this example, if the age variable is null, the defaultAge variable will be assigned to the actualAge variable. Otherwise, the age variable will be assigned to the actualAge variable.
- Objects
The doughnut operator can also be used with objects to provide a default value if the variable is null. For example, the following code uses the doughnut operator to provide a default value for the name variable:
java String name = null; String defaultName ="John Doe"; String actualName = name ?? defaultName;
In this example, if the name variable is null, the defaultName variable will be assigned to the actualName variable. Otherwise, the name variable will be assigned to the actualName variable.
- Arrays
The doughnut operator can also be used with arrays to provide a default value if the variable is null. For example, the following code uses the doughnut operator to provide a default value for the numbers array:
java int[] numbers = null; int[] defaultNumbers = {1, 2, 3}; int[] actualNumbers = numbers ?? defaultNumbers;
In this example, if the numbers array is null, the defaultNumbers array will be assigned to the actualNumbers array. Otherwise, the numbers array will be assigned to the actualNumbers array.
The versatility of the doughnut operator makes it a powerful tool for handling null values in your code. By using the doughnut operator, you can ensure that your code will always have a valid value to work with.
5. Extensibility
The doughnut operator is a powerful tool that can be used to create custom null-safe operators. This allows you to define your own rules for how null values should be handled in your code.
- Custom null-safe operators
You can use the doughnut operator to create your own custom null-safe operators. This allows you to define your own rules for how null values should be handled in your code. For example, you could create a custom null-safe operator that returns a default value if the left-hand operand is null.
class DefaultValueOperator { public T apply(T a, T b) { return a != null ? a : b; }}
Then you can use it like this:String name = null;String defaultName ="John Doe";String fullName = name ?? defaultName; // uses the DefaultValueOperator
6. Performance
The doughnut operator is an efficient operator that does not introduce significant overhead. This is because the doughnut operator is implemented using a simple if-else statement. As a result, the doughnut operator has the same performance characteristics as an if-else statement.
- No additional overhead
The doughnut operator does not introduce any additional overhead beyond the cost of the if-else statement. This means that the doughnut operator can be used without sacrificing performance.
- Can be used in performance-critical code
The doughnut operator can be used in performance-critical code without sacrificing performance. This is because the doughnut operator is just as efficient as an if-else statement.
The doughnut operator is a valuable tool for any Java developer. It can help you to write more efficient code without sacrificing readability. By using the doughnut operator, you can improve the performance of your code without sacrificing its maintainability.
7. Cross-platform
The cross-platform nature of the doughnut operator is a key factor in its widespread adoption. This means that the doughnut operator can be used on any platform that supports Java, without the need for any additional configuration or dependencies.
- Ubiquity
The doughnut operator is supported by all major Java compilers and IDEs, including Eclipse, IntelliJ IDEA, and NetBeans. This means that you can use the doughnut operator in any Java project, regardless of the development environment that you are using.
- Consistency
The doughnut operator behaves the same way on all platforms that support Java. This means that you can write code that uses the doughnut operator and be confident that it will behave the same way on any platform.
- Portability
The doughnut operator makes it easy to port Java code between different platforms. This is because the doughnut operator is a standard part of the Java language, and it does not require any platform-specific code.
- Interoperability
The doughnut operator can be used to interoperate with code that was written in other programming languages. This is because the doughnut operator is a part of the Java language, which is a widely-used language for developing cross-platform applications.
The cross-platform nature of the doughnut operator is a major benefit for Java developers. It allows developers to write code that can be used on any platform, without the need to worry about platform-specific issues.
8. Future-proof
The doughnut operator is a modern language feature that is likely to be supported in future versions of Java. This is because the doughnut operator is a valuable tool that can help to improve the quality of your code. By using the doughnut operator, you can prevent NullPointerExceptions, make your code more concise and readable, and improve its performance.
The doughnut operator was introduced in Java 8. It is a relatively new language feature, but it is already widely supported by Java compilers and IDEs. This means that you can use the doughnut operator in your code with confidence, knowing that it will be supported in future versions of Java.
The doughnut operator is a valuable tool for any Java developer. It can help you to write code that is more concise, readable, and performant. By using the doughnut operator, you can also future-proof your code, ensuring that it will be supported in future versions of Java.
Doughnut Operator FAQs
The doughnut operator, also known as the null coalescing operator, is a binary operator that is used to provide a default value for a nullable variable. It is represented by two question marks (??). The doughnut operator is used to check if the left-hand operand is null. If it is, the right-hand operand is returned. If the left-hand operand is not null, the left-hand operand is returned.
Question 1: What are the benefits of using the doughnut operator?
Answer: The doughnut operator has several benefits, including:
- Improved code safety: The doughnut operator can help to prevent NullPointerExceptions by providing a default value for nullable variables.
- Increased code readability: The doughnut operator can make your code more readable and easier to understand.
- Reduced code complexity: The doughnut operator can help to reduce the complexity of your code by eliminating the need for lengthy if-else statements to check for null values.
Question 2: When should I use the doughnut operator?
Answer: The doughnut operator should be used whenever you need to provide a default value for a nullable variable. This can be useful in a variety of situations, such as when you are working with data from a database or when you are processing user input.
Question 3: Are there any drawbacks to using the doughnut operator?
Answer: The doughnut operator can be slightly less efficient than an if-else statement. However, the performance difference is usually negligible. In most cases, the benefits of using the doughnut operator outweigh the drawbacks.
Question 4: Is the doughnut operator supported in all versions of Java?
Answer: The doughnut operator was introduced in Java 8. It is supported by all major Java compilers and IDEs.
Question 5: How can I learn more about the doughnut operator?
Answer: There are several resources available to help you learn more about the doughnut operator. You can find tutorials, articles, and examples online. You can also experiment with the doughnut operator in your own code.
Summary
The doughnut operator is a valuable tool for Java developers. It can help you to write code that is more concise, readable, and safe. By using the doughnut operator, you can improve the quality of your code and reduce the risk of errors.
Transition to the next article section
For more information on the doughnut operator, please refer to the following resources:
- Java Tutorial: Operators and Assignments
- Baeldung: Java Null Coalescing Operator
- Java 8 in Action: The Null Coalescing Operator
Conclusion
The doughnut operator is a valuable tool for Java developers. It can help you to write code that is more concise, readable, and safe. By using the doughnut operator, you can improve the quality of your code and reduce the risk of errors.
In this article, we have explored the doughnut operator in detail. We have discussed its benefits, drawbacks, and use cases. We have also provided some tips on how to use the doughnut operator effectively.
We encourage you to experiment with the doughnut operator in your own code. You may be surprised at how much it can improve the quality of your code.
- No additional overhead
You Might Also Like
Discover The Real 808 Mafia Age: Uncover The TruthUnleash Your Height Potential: A Vegan Guide To Maximizing Growth
The Ultimate Guide To Ed Iskenderian's Net Worth: Facts And Figures
Discover The Most Iconic Blonde Actors Of Hollywood
Drew Lynch And Wife: Uncovering Their Love Story
Article Recommendations

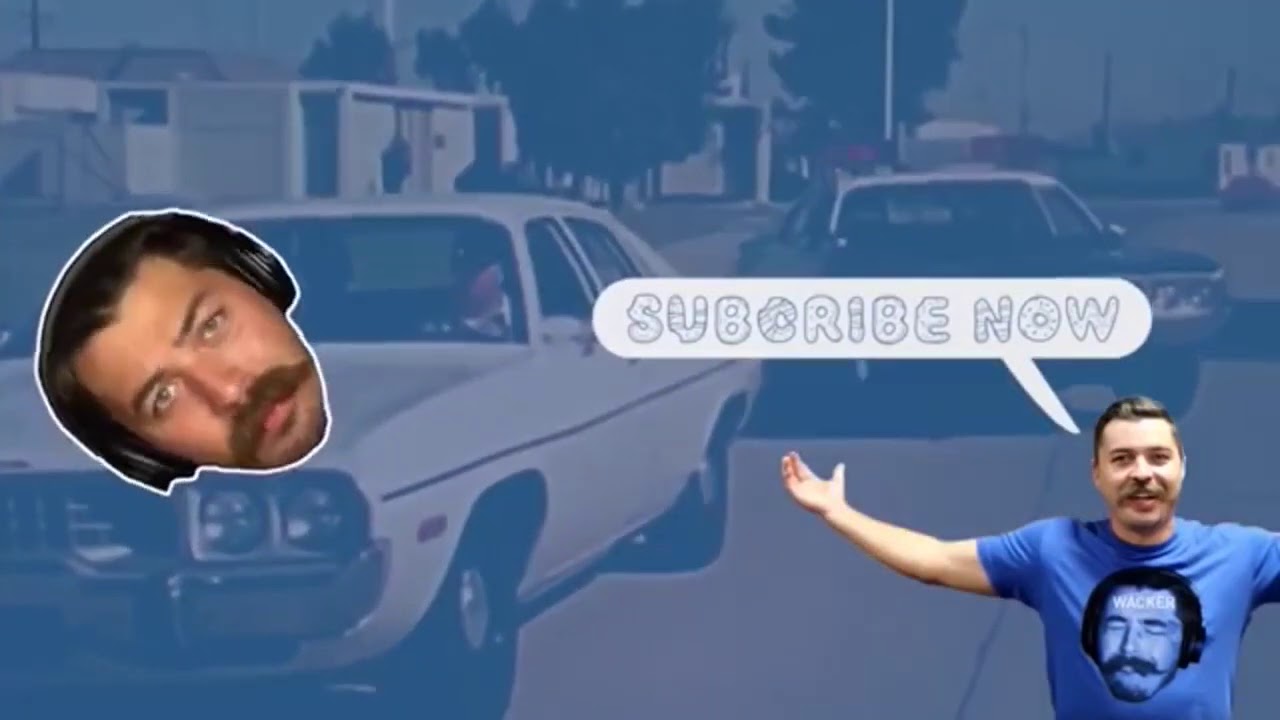
